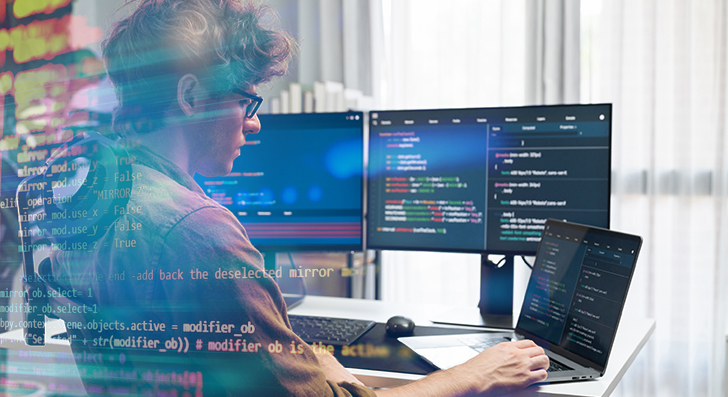
Scalability signifies your application can tackle advancement—far more consumers, additional knowledge, and a lot more visitors—without breaking. To be a developer, constructing with scalability in mind will save time and tension later on. Here’s a transparent and sensible tutorial that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not a little something you bolt on later on—it ought to be element within your prepare from the start. Several purposes fall short every time they improve quick for the reason that the original design can’t take care of the additional load. As being a developer, you'll want to Believe early regarding how your program will behave stressed.
Start by planning your architecture to generally be flexible. Prevent monolithic codebases where almost everything is tightly related. Alternatively, use modular structure or microservices. These patterns split your application into lesser, independent elements. Just about every module or service can scale By itself without the need of affecting The entire technique.
Also, give thought to your database from day a single. Will it will need to take care of 1,000,000 end users or merely 100? Pick the correct variety—relational or NoSQL—based upon how your details will grow. Strategy for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional essential level is in order to avoid hardcoding assumptions. Don’t publish code that only will work underneath present-day circumstances. Take into consideration what would come about When your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use design styles that assistance scaling, like message queues or event-driven systems. These assistance your application cope with additional requests devoid of receiving overloaded.
If you Make with scalability in your mind, you're not just getting ready for success—you happen to be lessening upcoming problems. A properly-planned procedure is less complicated to keep up, adapt, and expand. It’s much better to organize early than to rebuild later.
Use the ideal Databases
Selecting the correct database is a vital Component of constructing scalable programs. Not all databases are constructed the same, and utilizing the Mistaken one can gradual you down or simply induce failures as your application grows.
Commence by comprehending your details. Could it be extremely structured, like rows inside of a desk? If Sure, a relational databases like PostgreSQL or MySQL is a superb in shape. They're powerful with interactions, transactions, and consistency. In addition they help scaling tactics like go through replicas, indexing, and partitioning to take care of more website traffic and information.
In the event your info is a lot more flexible—like person activity logs, product or service catalogs, or documents—take into account a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and will scale horizontally much more quickly.
Also, consider your read through and write patterns. Will you be undertaking many reads with fewer writes? Use caching and browse replicas. Will you be handling a large publish load? Take a look at databases that will take care of superior publish throughput, or even occasion-based mostly facts storage systems like Apache Kafka (for short-term info streams).
It’s also clever to Imagine ahead. You may not require Highly developed scaling features now, but choosing a database that supports them implies you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your info dependant upon your entry designs. And constantly watch databases effectiveness when you improve.
Briefly, the appropriate databases depends on your application’s composition, velocity desires, And just how you assume it to increase. Just take time to choose properly—it’ll conserve lots of difficulty later.
Optimize Code and Queries
Quick code is key to scalability. As your application grows, just about every modest delay adds up. Improperly penned code or unoptimized queries can decelerate functionality and overload your process. That’s why it’s essential to Create effective logic from the start.
Get started by producing thoroughly clean, easy code. Avoid repeating logic and take away anything at all pointless. Don’t pick the most complex Option if an easy one is effective. Maintain your functions small, targeted, and straightforward to check. Use profiling tools to uncover bottlenecks—sites the place your code requires much too prolonged to run or works by using a lot of memory.
Future, have a look at your database queries. These typically slow factors down a lot more than the code itself. Be certain Each and every question only asks for the data you truly require. Prevent Choose *, which fetches anything, and rather pick out particular fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, In particular across huge tables.
For those who discover the exact same data getting asked for again and again, use caching. Retailer the results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application much more productive.
Make sure to take a look at with significant datasets. Code and queries that function fantastic with one hundred data could crash every time they have to handle 1 million.
In short, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These methods enable your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of a lot more consumers and a lot more targeted traffic. If anything goes by just one server, it can immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two resources assist keep your app quickly, stable, and scalable.
Load balancing spreads incoming traffic throughout various servers. In place of just one server undertaking every one of the perform, the load balancer routes customers to different servers based on availability. This suggests no solitary server receives overloaded. If one particular server goes down, the load balancer can deliver visitors to the Some others. Instruments like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info temporarily here so it might be reused speedily. When customers ask for the same information and facts all over again—like a product web page or simply a profile—you don’t ought to fetch it in the databases whenever. You are able to provide it from your cache.
There's two widespread kinds of caching:
one. Server-aspect caching (like Redis or Memcached) stores facts in memory for quickly obtain.
2. Shopper-side caching (like browser caching or CDN caching) outlets static information near the user.
Caching lessens database load, enhances velocity, and can make your application a lot more economical.
Use caching for things that don’t improve usually. And normally ensure your cache is current when information does transform.
In short, load balancing and caching are uncomplicated but powerful equipment. Alongside one another, they help your app deal with additional users, remain speedy, and recover from troubles. If you propose to develop, you require both.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need instruments that permit your app grow very easily. That’s the place cloud platforms and containers are available. They give you flexibility, minimize setup time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and expert services as you would like them. You don’t have to purchase hardware or guess long run potential. When targeted visitors increases, you are able to include a lot more assets with only a few clicks or routinely employing car-scaling. When website traffic drops, you may scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection equipment. It is possible to deal with setting up your application in place of taking care of infrastructure.
Containers are One more essential Device. A container packages your application and all the things it ought to operate—code, libraries, settings—into 1 device. This can make it effortless to move your application between environments, from a laptop computer for the cloud, with out surprises. Docker is the most popular Resource for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part within your app crashes, it restarts it immediately.
Containers also make it straightforward to individual elements of your application into providers. You are able to update or scale pieces independently, which can be perfect for functionality and reliability.
To put it briefly, making use of cloud and container tools signifies you are able to scale rapid, deploy very easily, and Get better swiftly when complications take place. If you'd like your application to develop without the need of limitations, start out utilizing these instruments early. They save time, lessen risk, and enable you to continue to be focused on constructing, not correcting.
Keep track of Anything
If you don’t keep an eye on your software, you won’t know when items go wrong. Monitoring can help the thing is how your app is executing, place challenges early, and make much better selections as your application grows. It’s a key A part of constructing scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These tell you how your servers and solutions are undertaking. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this knowledge.
Don’t just watch your servers—observe your application too. Keep an eye on how long it will take for consumers to load webpages, how often problems come about, and the place they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Arrange alerts for vital complications. As an example, Should your response time goes over a limit or a service goes down, you should get notified immediately. This allows you take care of challenges rapid, generally ahead of end users even recognize.
Monitoring is also practical any time you make improvements. In case you deploy a fresh function and find out a spike in glitches or slowdowns, you could roll it back again just before it causes serious problems.
As your app grows, traffic and details enhance. With out checking, you’ll overlook signs of hassle right up until it’s as well late. But with the ideal instruments in place, you continue to be in control.
To put it briefly, monitoring helps you maintain your app trusted and scalable. It’s not nearly recognizing failures—it’s about knowledge your program and ensuring that it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large providers. Even tiny applications require a solid foundation. By planning carefully, optimizing correctly, and utilizing the proper applications, you'll be able to Make apps that increase effortlessly without having breaking stressed. Start modest, Imagine major, and build wise.